Note
Click here to download the full example code
06.a sns.heatmap
for CRI v1
Plot rectangular data as a color-encoded matrix.
The generates a heatmap visualization for a dataset related to collateral sensitivity. It uses the Seaborn library to plot the rectangular data as a color-encoded matrix. The code loads the data from a CSV file, creates mappings for categories and colors, and then plots the heatmap using the loaded data and color maps. It also includes annotations, colorbar axes, category patches, legend elements, and formatting options to enhance the visualization.
16 # Libraries
17 import numpy as np
18 import pandas as pd
19 import seaborn as sns
20 import matplotlib as mpl
21 import matplotlib.pyplot as plt
22
23 from pathlib import Path
24 from matplotlib.patches import Patch
25 from matplotlib.colors import LogNorm
26 from matplotlib.patches import Rectangle
27
28 # See https://matplotlib.org/devdocs/users/explain/customizing.html
29 mpl.rcParams['axes.titlesize'] = 8
30 mpl.rcParams['axes.labelsize'] = 8
31 mpl.rcParams['xtick.labelsize'] = 8
32 mpl.rcParams['ytick.labelsize'] = 8
33
34 try:
35 __file__
36 TERMINAL = True
37 except:
38 TERMINAL = False
Lets load the data and create color mapping variables
45 # Load data
46 path = Path('../../datasets/collateral-sensitivity/sample')
47 data = pd.read_csv(path / 'matrix.csv', index_col=0)
48 abxs = pd.read_csv(path / 'categories.csv', index_col=0)
49
50 # Create dictionary to map category to color
51 labels = abxs.category
52 palette = sns.color_palette('colorblind', labels.nunique())
53 palette = sns.cubehelix_palette(labels.nunique(),
54 light=.9, dark=.1, reverse=True, start=1, rot=-2)
55 lookup = dict(zip(labels.unique(), palette))
56
57 # Create dictionary to map code to category
58 code2cat = dict(zip(abxs.antimicrobial_code, abxs.category))
59
60 # Create colors
61 colors = data.columns.to_series().map(code2cat).map(lookup)
Lets see the data
66 if TERMINAL:
67 print("\nData:")
68 print(data)
69 data.iloc[:7,:7]
Lets see the antimicrobials
73 if TERMINAL:
74 print("\nAntimicrobials:")
75 print(abxs)
76 abxs
Lets create some variables.
82 # Create color maps
83 cmapu = sns.color_palette("YlGn", as_cmap=True)
84 cmapl = sns.diverging_palette(220, 20, as_cmap=True)
85
86 # Create triangular matrices
87 masku = np.triu(np.ones_like(data))
88 maskl = np.tril(np.ones_like(data))
Let’s display a heatmap
95 # Draw (heatmap)
96 fig, axs = plt.subplots(nrows=1, ncols=1,
97 sharey=False, sharex=False, figsize=(8, 5)
98 )
99
100 # .. note: This is used to create the colorbar axes. If we want
101 # the default display in which the use the whole fig
102 # height just pass cbar_ax=None.
103
104 # Create own colorbar axes
105 # Params are [left, bottom, width, height]
106 cbar_ax1 = fig.add_axes([0.76, 0.5, 0.03, 0.38])
107 cbar_ax2 = fig.add_axes([0.90, 0.5, 0.03, 0.38])
108
109 # Display
110 r1 = sns.heatmap(data=data, cmap=cmapu, mask=masku, ax=axs,
111 annot=False, linewidth=0.5, norm=LogNorm(),
112 annot_kws={"size": 8}, square=True, vmin=0,
113 cbar_ax=cbar_ax2,
114 cbar_kws={'label': 'Number of isolates'})
115
116 r2 = sns.heatmap(data=data, cmap=cmapl, mask=maskl, ax=axs,
117 annot=False, linewidth=0.5, vmin=-0.7, vmax=0.7,
118 center=0, annot_kws={"size": 8}, square=True,
119 xticklabels=True, yticklabels=True,
120 cbar_ax=cbar_ax1,
121 cbar_kws={'label': 'Collateral Resistance Index'})
122
123 # Create patches for categories
124 category_patches = []
125 for i in axs.get_xticklabels():
126 try:
127 x, y = i.get_position()
128 c = colors.to_dict().get(i.get_text(), 'k')
129 #i.set_color(c) # for testing
130
131 # Add patch.
132 category_patches.append(
133 Rectangle((x-0.35, y-0.5), 0.8, 0.3, edgecolor='k',
134 facecolor=c, fill=True, lw=0.25, alpha=0.5, zorder=1000,
135 transform=axs.transData
136 )
137 )
138 except Exception as e:
139 print(i.get_text(), e)
140
141 # Add category rectangles
142 fig.patches.extend(category_patches)
143
144 # Create legend elements
145 legend_elements = [
146 Patch(facecolor=v, edgecolor='k',
147 fill=True, lw=0.25, alpha=0.5, label=k)
148 for k, v in lookup.items()
149 ]
150
151 # Add legend
152 axs.legend(handles=legend_elements, loc='lower left',
153 ncol=1, bbox_to_anchor=(1.1, 0.1), fontsize=8,
154 fancybox=False, shadow=False)
155
156 # Format
157 plt.suptitle('URINE - Escherichia Coli')
158 #plt.subplots_adjust(left=0.8)
159 plt.tight_layout()
160
161 # Show
162 plt.show()
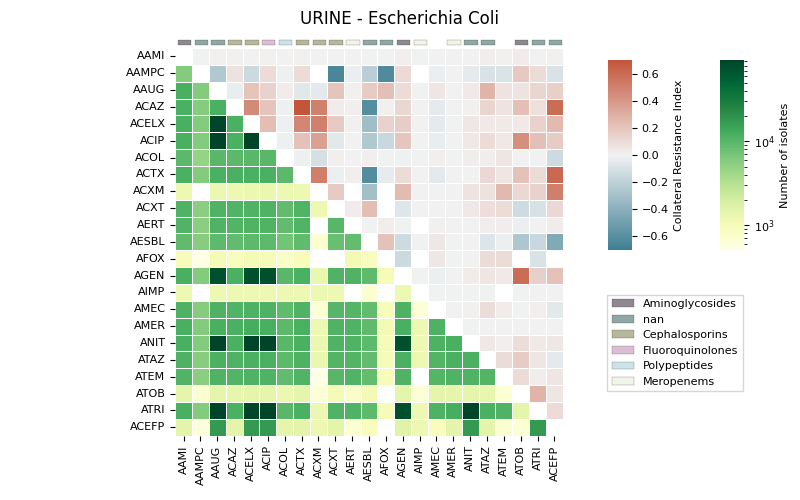
Out:
AMEC Invalid RGBA argument: nan
ATEM Invalid RGBA argument: nan
/Users/cbit/Desktop/repositories/github/python-spare-code/main/examples/matplotlib/plot_main06_a_heatmap.py:159: UserWarning:
This figure includes Axes that are not compatible with tight_layout, so results might be incorrect.
Let’s draw a clustermap
168 """
169 # Display
170 result = sns.clustermap(data=data, figsize=(6,6),
171 cmap=cmapu, mask=masku, annot=False, linewidth=0.5,
172 norm=LogNorm(), annot_kws={"size": 8}, square=True, vmin=0,
173 row_cluster=False, col_cluster=False,
174 col_colors=colors,
175 xticklabels=True, yticklabels=True)
176
177 #sns.heatmap(data=data, cmap=cmapl, mask=maskl, ax=result.ax_heatmap,
178 # annot=False, linewidth=0.5, vmin=-0.7, vmax=0.7,
179 # center=0, annot_kws={"size": 8}, square=True,
180 # xticklabels=False, yticklabels=False)
181
182 # Move the colorbar to the empty space.
183 #result.ax_col_dendrogram.legend(loc="center", ncol=6)
184 #result.cax.set_position([.15, .2, .03, .45])
185 """
Out:
'\n# Display\nresult = sns.clustermap(data=data, figsize=(6,6),\n cmap=cmapu, mask=masku, annot=False, linewidth=0.5,\n norm=LogNorm(), annot_kws={"size": 8}, square=True, vmin=0,\n row_cluster=False, col_cluster=False,\n col_colors=colors,\n xticklabels=True, yticklabels=True)\n\n#sns.heatmap(data=data, cmap=cmapl, mask=maskl, ax=result.ax_heatmap,\n# annot=False, linewidth=0.5, vmin=-0.7, vmax=0.7,\n# center=0, annot_kws={"size": 8}, square=True,\n# xticklabels=False, yticklabels=False)\n\n# Move the colorbar to the empty space.\n#result.ax_col_dendrogram.legend(loc="center", ncol=6)\n#result.cax.set_position([.15, .2, .03, .45])\n'
Total running time of the script: ( 0 minutes 0.539 seconds)