Note
Click here to download the full example code
02. mpl.hexbin
Make a 2D hexagonal binning plot of points x, y.
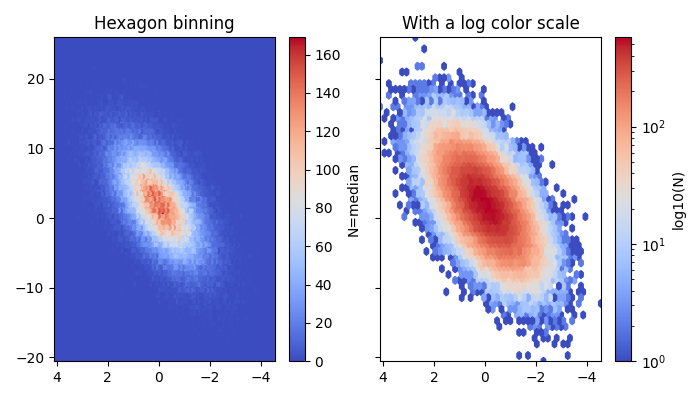
9 import pandas as pd
10 import numpy as np
11 import matplotlib.pyplot as plt
12
13 # constant
14 n = 100000
15
16 # Fixing random state for reproducibility
17 np.random.seed(19680801)
18
19 # Generate data
20 x = np.random.standard_normal(n)
21 y = 2.0 + 3.0 * x + 4.0 * np.random.standard_normal(n)
22 z = None
23
24 # Compute limits
25 xmin = x.min()
26 xmax = x.max()
27 ymin = y.min()
28 ymax = y.max()
29
30 # Display hexagon binning (linear)
31 fig, axs = plt.subplots(ncols=2, sharey=True, figsize=(7, 4))
32 fig.subplots_adjust(hspace=0.5, left=0.07, right=0.93)
33 ax = axs[0]
34 hb = ax.hexbin(x, y, C=z, cmap='coolwarm', reduce_C_function=np.median)
35 ax.axis([xmin, xmax, ymin, ymax])
36 ax.set_title("Hexagon binning")
37 ax.invert_xaxis()
38 cb = fig.colorbar(hb, ax=ax)
39 cb.set_label('N=median')
40
41 # Display hexagon binning (log)
42 ax = axs[1]
43 hb = ax.hexbin(x, y, C=z, gridsize=50,
44 bins='log', cmap='coolwarm',
45 reduce_C_function=np.median)
46 ax.axis([xmin, xmax, ymin, ymax])
47 ax.set_title("With a log color scale")
48 ax.invert_xaxis()
49 cb = fig.colorbar(hb, ax=ax)
50 cb.set_label('log10(N)')
51
52 # Show
53 plt.tight_layout()
54 plt.show()
Total running time of the script: ( 0 minutes 0.853 seconds)