Note
Go to the end to download the full example code
SART
- Trend as slope of WLS
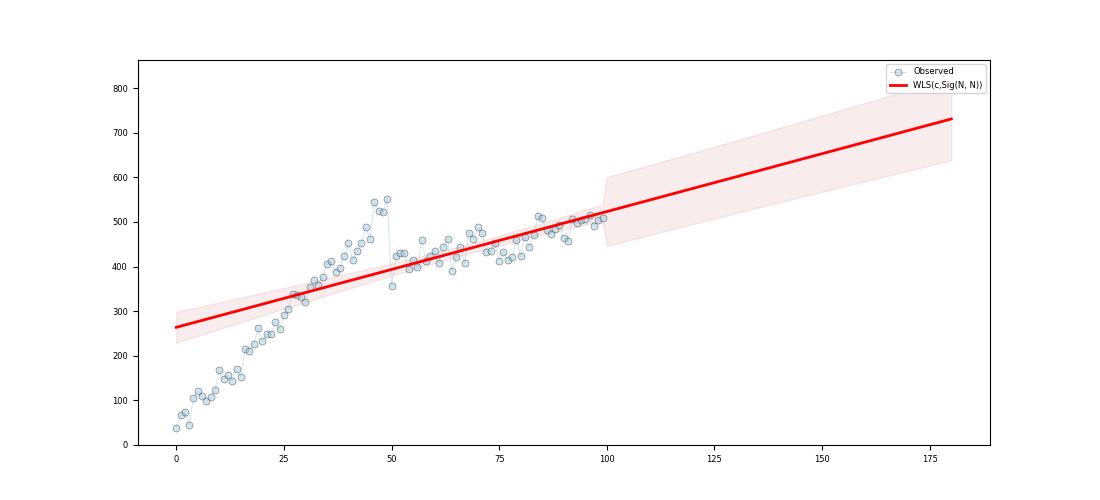
c:\users\kelda\desktop\repositories\virtualenvs\venv-py391-pyamr\lib\site-packages\statsmodels\regression\linear_model.py:807: RuntimeWarning:
divide by zero encountered in log
Series:
wls-rsquared 0.5402
wls-rsquared_adj 0.5355
wls-fvalue 115.1527
wls-fprob 0.0
wls-aic inf
wls-bic inf
wls-llf -inf
wls-mse_model 165533.2802
wls-mse_resid 1437.5111
wls-mse_total 3095.0441
wls-const_coef 263.575
wls-const_std 17.4154
wls-const_tvalue 15.1346
wls-const_tprob 0.0
wls-const_cil 229.0148
wls-const_ciu 298.1352
wls-x1_coef 2.5975
wls-x1_std 0.2421
wls-x1_tvalue 10.7309
wls-x1_tprob 0.0
wls-x1_cil 2.1171
wls-x1_ciu 3.0778
wls-s_dw 0.546
wls-s_jb_value 14.895
wls-s_jb_prob 0.0006
wls-s_skew 0.697
wls-s_kurtosis 4.278
wls-s_omnibus_value 12.662
wls-s_omnibus_prob 0.002
wls-m_dw 0.1707
wls-m_jb_value 4.7339
wls-m_jb_prob 0.0938
wls-m_skew -0.5009
wls-m_kurtosis 3.364
wls-m_nm_value 5.3656
wls-m_nm_prob 0.0684
wls-m_ks_value 0.5809
wls-m_ks_prob 0.0
wls-m_shp_value 0.9455
wls-m_shp_prob 0.0004
wls-m_ad_value 2.4183
wls-m_ad_nnorm False
wls-missing raise
wls-exog [[1.0, 0.0...
wls-endog [38.780154...
wls-trend c
wls-weights [0.0328805...
wls-W <pyamr.met...
wls-model <statsmode...
wls-id WLS(c,Sig(...
dtype: object
Regression line:
[263.58 266.17 268.77 271.37 273.96 276.56 279.16 281.76 284.35 286.95]
Summary:
WLS Regression Results
==============================================================================
Dep. Variable: y R-squared: 0.540
Model: WLS Adj. R-squared: 0.536
Method: Least Squares F-statistic: 115.2
Date: Thu, 15 Jun 2023 Prob (F-statistic): 3.16e-18
Time: 18:17:11 Log-Likelihood: -inf
No. Observations: 100 AIC: inf
Df Residuals: 98 BIC: inf
Df Model: 1
Covariance Type: nonrobust
==============================================================================
coef std err t P>|t| [0.025 0.975]
------------------------------------------------------------------------------
const 263.5750 17.415 15.135 0.000 229.015 298.135
x1 2.5975 0.242 10.731 0.000 2.117 3.078
==============================================================================
Omnibus: 12.662 Durbin-Watson: 0.546
Prob(Omnibus): 0.002 Jarque-Bera (JB): 14.895
Skew: 0.697 Prob(JB): 0.000583
Kurtosis: 4.278 Cond. No. 231.
Normal (N): 5.366 Prob(N): 0.068
==============================================================================
14 # Import class.
15 import sys
16 import numpy as np
17 import pandas as pd
18 import matplotlib as mpl
19 import matplotlib.pyplot as plt
20 import statsmodels.api as sm
21 import statsmodels.robust.norms as norms
22
23 # import weights.
24 from pyamr.datasets.load import make_timeseries
25 from pyamr.core.regression.wls import WLSWrapper
26 from pyamr.metrics.weights import SigmoidA
27
28 # ----------------------------
29 # set basic configuration
30 # ----------------------------
31 # Matplotlib options
32 mpl.rc('legend', fontsize=6)
33 mpl.rc('xtick', labelsize=6)
34 mpl.rc('ytick', labelsize=6)
35
36 # Set pandas configuration.
37 pd.set_option('display.max_colwidth', 14)
38 pd.set_option('display.width', 150)
39 pd.set_option('display.precision', 4)
40
41 # ----------------------------
42 # create data
43 # ----------------------------
44 # Create timeseries data
45 x, y, f = make_timeseries()
46
47 # Create method to compute weights from frequencies
48 W = SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0)
49
50 # Note that the function fit will call M.weights(weights) inside and will
51 # store the M converter in the instance. Therefore, the code executed is
52 # equivalent to <weights=M.weights(f)> with the only difference being that
53 # the weight converter is not saved.
54 wls = WLSWrapper(estimator=sm.WLS).fit( \
55 exog=x, endog=y, trend='c', weights=f,
56 W=W, missing='raise')
57
58 # Print series.
59 print("\nSeries:")
60 print(wls.as_series())
61
62 # Print regression line.
63 print("\nRegression line:")
64 print(wls.line(np.arange(10)))
65
66 # Print summary.
67 print("\nSummary:")
68 print(wls.as_summary())
69
70 # -----------------
71 # Save & Load
72 # -----------------
73 # File location
74 #fname = '../../examples/saved/wls-sample.pickle'
75
76 # Save
77 #wls.save(fname=fname)
78
79 # Load
80 #wls = WLSWrapper().load(fname=fname)
81
82 # -------------
83 # Example I
84 # -------------
85 # This example shows how to make predictions using the wrapper and how
86 # to plot the resultin data. In addition, it compares the intervales
87 # provided by get_prediction (confidence intervals) and the intervals
88 # provided by wls_prediction_std (prediction intervals).
89 #
90 # To Do: Implement methods to compute CI and PI (see regression).
91
92 # Variables.
93 start, end = None, 180
94
95 # Compute predictions (exogenous?). It returns a 2D array
96 # where the rows contain the time (t), the mean, the lower
97 # and upper confidence (or prediction?) interval.
98 preds = wls.get_prediction(start=start, end=end)
99
100
101 # Create figure
102 fig, ax = plt.subplots(1, 1, figsize=(11,5))
103
104 # Plotting confidence intervals
105 # -----------------------------
106 # Plot truth values.
107 ax.plot(x, y, color='#A6CEE3', alpha=0.5, marker='o',
108 markeredgecolor='k', markeredgewidth=0.5,
109 markersize=5, linewidth=0.75, label='Observed')
110
111 # Plot forecasted values.
112 ax.plot(preds[0,:], preds[1, :], color='#FF0000', alpha=1.00,
113 linewidth=2.0, label=wls._identifier(short=True))
114
115 # Plot the confidence intervals.
116 ax.fill_between(preds[0, :], preds[2, :],
117 preds[3, :],
118 color='r',
119 alpha=0.1)
120
121 # Legend
122 plt.legend()
123
124 # Show
125 plt.show()
Total running time of the script: ( 0 minutes 0.108 seconds)