Note
Go to the end to download the full example code
Table Scatterplot
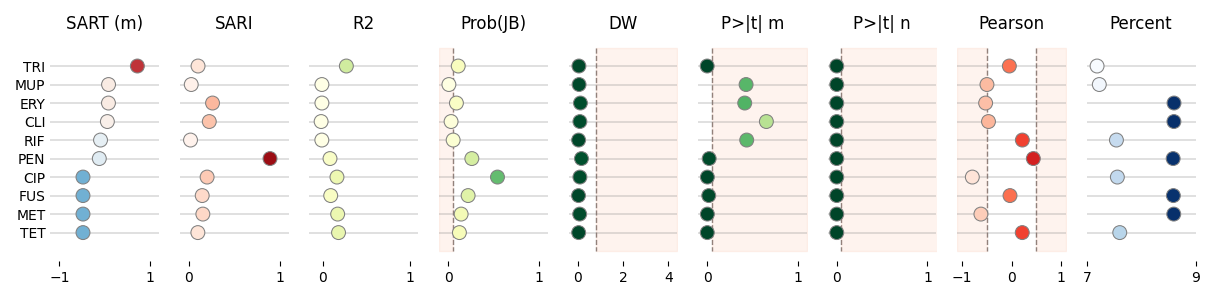
Data:
idx org abx sart_m sari r2 jb dw ptm ptn pearson percent
0 9 SAUR TRI 0.720 0.100 0.274 0.112 0.056 0.000 0.0 -0.046 7.189
1 8 SAUR MUP 0.084 0.025 -0.007 0.009 0.064 0.429 0.0 -0.499 7.230
2 7 SAUR ERY 0.082 0.260 -0.007 0.092 0.123 0.414 0.0 -0.526 8.604
3 6 SAUR CLI 0.058 0.224 -0.016 0.033 0.099 0.653 0.0 -0.468 8.602
4 5 SAUR RIF -0.093 0.017 -0.008 0.056 0.043 0.436 0.0 0.217 7.545
5 4 SAUR PEN -0.120 0.894 0.086 0.262 0.168 0.022 0.0 0.439 8.588
6 0 SAUR CIP -0.480 0.200 0.166 0.545 0.100 0.002 0.0 -0.795 7.562
7 1 SAUR FUS -0.480 0.146 0.094 0.221 0.038 0.017 0.0 -0.033 8.592
8 2 SAUR MET -0.480 0.153 0.174 0.144 0.090 0.002 0.0 -0.621 8.599
9 3 SAUR TET -0.480 0.098 0.184 0.125 0.045 0.001 0.0 0.215 7.606
6 # Libraries
7 import numpy as np
8 import pandas as pd
9 import seaborn as sns
10 import matplotlib.pyplot as plt
11 import matplotlib.gridspec as gridspec
12
13 from matplotlib import colors
14
15 # Own libraries
16 from pyamr.utils.plot import MidpointNormalize
17 from pyamr.utils.plot import vlinebgplot
18 from pyamr.graphics.table_graph import _DEFAULT_CONFIGURATION
19
20 # -------------------------------------------
21 # Main
22 # -------------------------------------------
23
24 # Create data
25 data = [
26 [9, 'SAUR', 'TRI', 0.720, 0.100, 0.274, 0.112, 0.056, 0.000, 0.0, -0.046, 7.189],
27 [8, 'SAUR', 'MUP', 0.084, 0.025, -0.007, 0.009, 0.064, 0.429, 0.0, -0.499, 7.230],
28 [7, 'SAUR', 'ERY', 0.082, 0.260, -0.007, 0.092, 0.123, 0.414, 0.0, -0.526, 8.604],
29 [6, 'SAUR', 'CLI', 0.058, 0.224, -0.016, 0.033, 0.099, 0.653, 0.0, -0.468, 8.602],
30 [5, 'SAUR', 'RIF', -0.093, 0.017, -0.008, 0.056, 0.043, 0.436, 0.0, 0.217, 7.545],
31 [4, 'SAUR', 'PEN', -0.120, 0.894, 0.086, 0.262, 0.168, 0.022, 0.0, 0.439, 8.588],
32 [0, 'SAUR', 'CIP', -0.480, 0.200, 0.166, 0.545, 0.100, 0.002, 0.0, -0.795, 7.562],
33 [1, 'SAUR', 'FUS', -0.480, 0.146, 0.094, 0.221, 0.038, 0.017, 0.0, -0.033, 8.592],
34 [2, 'SAUR', 'MET', -0.480, 0.153, 0.174, 0.144, 0.090, 0.002, 0.0, -0.621, 8.599],
35 [3, 'SAUR', 'TET', -0.480, 0.098, 0.184, 0.125, 0.045, 0.001, 0.0, 0.215, 7.606]
36 ]
37
38 # Create dataframe
39 data = pd.DataFrame(data, columns=['idx', 'org', 'abx',
40 'sart_m', 'sari', 'r2', 'jb', 'dw', 'ptm', 'ptn',
41 'pearson', 'percent'])
42
43 # Show
44 print("\nData:")
45 print(data)
46
47 # Filter features
48 data = data[data.columns[2:]]
49
50 # -------------------------------------------
51 # Pair Grid
52 # -------------------------------------------
53 # Create plotting configuration
54 info = _DEFAULT_CONFIGURATION
55 info['percent'] = {
56 'name': 'percent',
57 'cmap': 'Blues',
58 'title': 'Percent',
59 'xlim': [7, 9],
60 'xticks': [7, 9],
61 'vline': [],
62 }
63
64 # Create pair grid
65 g = sns.PairGrid(data, x_vars=data.columns[1:],
66 y_vars=["abx"], height=3, aspect=.45)
67
68 # Draw a dot plot using the stripplot function
69 # g.map(sns.stripplot, size=10, orient="h", jitter=False,
70 # palette="flare_r", linewidth=1, edgecolor="w")
71
72 # Set common features
73 g.set(xlabel='', ylabel='')
74
75 # Plot strips and format axes
76 for ax, c in zip(g.axes.flat, data.columns[1:]):
77
78 # Get information
79 d = info[c] if c in info else {}
80
81 # Display
82 # sns.stripplot(data=data, x=title, y='abx', size=10,
83 # orient="h", jitter=False, linewidth=0.75, ax=ax,
84 # edgecolor="gray", palette=d.get('cmap', None))
85 # color='b')
86
87 # .. note: We need to use scatter plot if we want to
88 # assign colors to the markers according to
89 # their value.
90
91 # Using scatter plot
92 sns.scatterplot(data=data, x=c, y='abx', s=100, alpha=1,
93 ax=ax, linewidth=0.75, edgecolor='gray',
94 c=data[c], cmap=d.get('cmap', None),
95 norm=d.get('norm', None))
96
97 # Plot vertical lines
98 for e in d.get('vline', []):
99 vlinebgplot(ax, top=data.shape[0], **e)
100
101 # Configure axes
102 ax.set(title=d.get('title', c),
103 xlim=d.get('xlim', None),
104 xticks=d.get('xticks', []),
105 xlabel='', ylabel='')
106 ax.tick_params(axis='y', which='both', length=0)
107 ax.xaxis.grid(False)
108 ax.yaxis.grid(visible=True, which='major',
109 color='gray', linestyle='-', linewidth=0.35)
110 ax.set_axisbelow(True)
111
112 # Despine
113 sns.despine(left=True, bottom=True)
114
115 # Adjust layout
116 plt.tight_layout()
117
118 # Show
119 plt.show()
Total running time of the script: ( 0 minutes 0.694 seconds)