Note
Go to the end to download the full example code
SARIMAX - Search
See SARIMAX
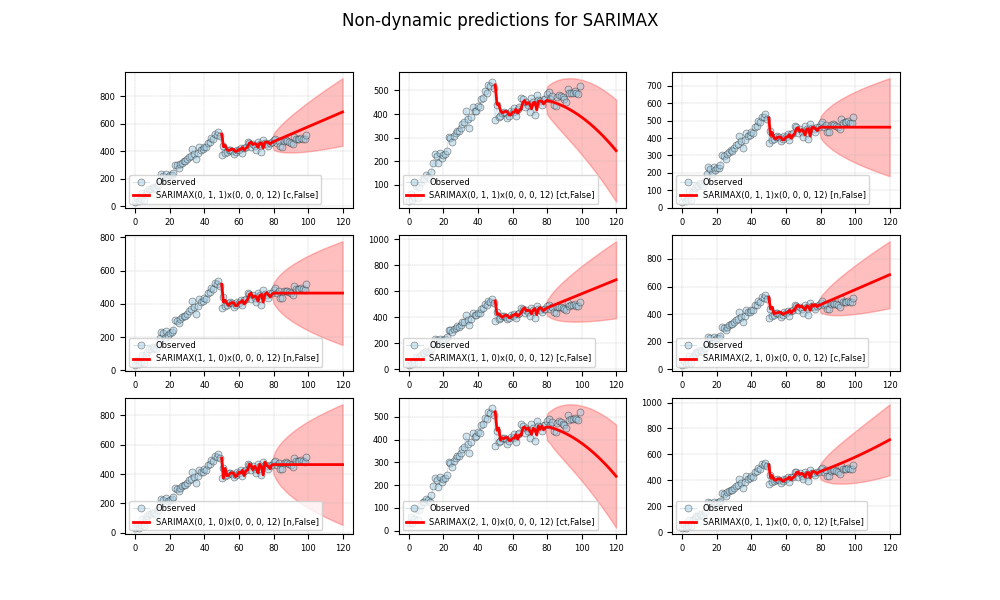
Summary:
sarimax-order sarimax-seasonal_order sarimax-trend sarimax-aic sarimax-bic
0 (0, 1, 1) (0, 0, 0, 12) c 769.3258 776.4342
1 (0, 1, 1) (0, 0, 0, 12) ct 767.6064 777.0842
2 (0, 1, 1) (0, 0, 0, 12) n 772.6176 777.3564
3 (1, 1, 0) (0, 0, 0, 12) n 773.3553 778.0942
4 (1, 1, 0) (0, 0, 0, 12) c 771.3858 778.4942
.. ... ... ... ... ...
287 (0, 0, 0) (0, 0, 0, 12) c 1018.8423 1023.6064
288 (0, 0, 2) (0, 0, 0, 12) n 1038.0866 1045.2327
289 (0, 0, 1) (0, 0, 0, 12) n 1073.558 1078.3221
290 (0, 0, 0) (0, 0, 1, 12) n 1103.419 1108.1831
291 (0, 0, 0) (0, 0, 0, 12) n 1172.8235 1175.2055
[292 rows x 5 columns]
0. Estimator (bic=776.43): SARIMAX(0, 1, 1)x(0, 0, 0, 12) [c,False]
1. Estimator (bic=777.08): SARIMAX(0, 1, 1)x(0, 0, 0, 12) [ct,False]
2. Estimator (bic=777.36): SARIMAX(0, 1, 1)x(0, 0, 0, 12) [n,False]
3. Estimator (bic=778.09): SARIMAX(1, 1, 0)x(0, 0, 0, 12) [n,False]
4. Estimator (bic=778.49): SARIMAX(1, 1, 0)x(0, 0, 0, 12) [c,False]
5. Estimator (bic=779.44): SARIMAX(2, 1, 0)x(0, 0, 0, 12) [c,False]
6. Estimator (bic=779.99): SARIMAX(0, 1, 0)x(0, 0, 0, 12) [n,False]
7. Estimator (bic=780.16): SARIMAX(2, 1, 0)x(0, 0, 0, 12) [ct,False]
8. Estimator (bic=780.46): SARIMAX(0, 1, 1)x(0, 0, 0, 12) [t,False]
8 # Import.
9 import sys
10 import warnings
11 import pandas as pd
12 import matplotlib as mpl
13 import matplotlib.pyplot as plt
14
15 # Import sarimax
16 from statsmodels.tsa.statespace.sarimax import SARIMAX
17
18 # import weights.
19 from pyamr.datasets.load import make_timeseries
20 from pyamr.core.regression.sarimax import SARIMAXWrapper
21
22 # Filter warnings
23 #warnings.simplefilter(action='ignore', category=FutureWarning)
24
25 # ----------------------------
26 # set basic configuration
27 # ----------------------------
28 # Matplotlib options
29 mpl.rc('legend', fontsize=6)
30 mpl.rc('xtick', labelsize=6)
31 mpl.rc('ytick', labelsize=6)
32
33 # Set pandas configuration.
34 pd.set_option('display.max_colwidth', 14)
35 pd.set_option('display.width', 150)
36 pd.set_option('display.precision', 4)
37
38 # ----------------------------
39 # create data
40 # ----------------------------
41 # Create timeseries data
42 x, y, f = make_timeseries()
43
44 # Create exogenous variable
45 exog = x
46
47 # Variables.
48 s, e = 50, 120
49
50 # -------------------------------
51 # create arima model
52 # -------------------------------
53 # This example shows how to use auto to find the best overall model using
54 # a particular seletion criteria. It also demonstrates how to plot the
55 # resulting data for visualization purposes. Note that it only prints
56 # the top best classifier according to the information criteria.
57
58 # Find the best arima model (bruteforce).
59 models, best = SARIMAXWrapper(estimator=SARIMAX) \
60 .auto(endog=y[:80], ic='bic',
61 max_ar=2,
62 max_ma=3,
63 max_d=1,
64 max_P=1,
65 max_D=0,
66 max_Q=1,
67 list_s=[12],
68 return_fits=True)
69
70 # Sort the list (from lower to upper)
71 models.sort(key=lambda x: x.bic, reverse=False)
72
73 # Summary
74 summary = SARIMAXWrapper().from_list_dataframe(models)
75
76 # Show summary
77 print("\nSummary:")
78 print(summary[['sarimax-order',
79 'sarimax-seasonal_order',
80 'sarimax-trend',
81 'sarimax-aic',
82 'sarimax-bic']])
83
84 # -------------------------------
85 # plot results
86 # -------------------------------
87 # Create figure
88 fig, axes = plt.subplots(3,3, figsize=(10,6))
89 axes = axes.flatten()
90
91 # Loop for the selected models
92 for i,estimator in enumerate(models[:9]):
93
94 # Show information
95 print("%2d. Estimator (bic=%.2f): %s " % \
96 (i, estimator.bic, estimator._identifier()))
97
98 # Get the predictions
99 preds = estimator.get_prediction(start=s, end=e, dynamic=False)
100
101 # Plot truth values.
102 axes[i].plot(y, color='#A6CEE3', alpha=0.5, marker='o',
103 markeredgecolor='k', markeredgewidth=0.5,
104 markersize=5, linewidth=0.75, label='Observed')
105
106 # Plot forecasted values.
107 axes[i].plot(preds[0,:], preds[1,:], color='#FF0000', alpha=1.00,
108 linewidth=2.0, label=estimator._identifier())
109
110 # Plot the confidence intervals.
111 axes[i].fill_between(preds[0,:], preds[2,:],
112 preds[3,:],
113 color='#FF0000',
114 alpha=0.25)
115
116 # Configure axes
117 axes[i].legend(loc=3)
118 axes[i].grid(True, linestyle='--', linewidth=0.25)
119
120 # Set superior title
121 plt.suptitle("Non-dynamic predictions for SARIMAX")
122
123 # Show
124 plt.show()
Total running time of the script: ( 1 minutes 50.105 seconds)