Note
Go to the end to download the full example code
SARIMAX - Basic
See SARIMAX
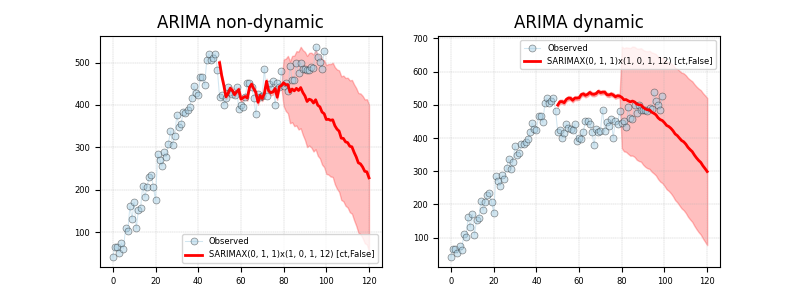
Series:
sarimax-aic 764.1138
sarimax-bic 778.3305
sarimax-hqic 769.8095
sarimax-llf -376.0569
sarimax-intercept_coef 23.9663
...
sarimax-seasonal_order (1, 0, 1, 12)
sarimax-order (0, 1, 1)
sarimax-disp 0
sarimax-model <statsmode...
sarimax-id SARIMAX(0,...
Length: 73, dtype: object
Summary:
SARIMAX Results
==========================================================================================
Dep. Variable: y No. Observations: 80
Model: SARIMAX(0, 1, 1)x(1, 0, 1, 12) Log Likelihood -376.057
Date: Thu, 15 Jun 2023 AIC 764.114
Time: 18:17:22 BIC 778.331
Sample: 0 HQIC 769.809
- 80
Covariance Type: opg
==============================================================================
coef std err z P>|z| [0.025 0.975]
------------------------------------------------------------------------------
intercept 23.9663 9.263 2.587 0.010 5.811 42.121
drift -0.3219 0.137 -2.345 0.019 -0.591 -0.053
ma.L1 -0.4928 0.089 -5.521 0.000 -0.668 -0.318
ar.S.L12 -0.7897 0.520 -1.518 0.129 -1.809 0.230
ma.S.L12 0.6418 0.639 1.005 0.315 -0.610 1.894
sigma2 784.2609 143.939 5.449 0.000 502.145 1066.377
==============================================================================
Ljung-Box(L1)(Q): 0.03 Jarque-Bera (JB): 0.69
Prob(Q): 0.87 Prob(JB): 0.71
Heteroskedasticity(H): 0.84 Skew: -0.23
Prob(H)(two-sided): 0.67 Kurtosis: 3.08
==============================================================================
Manual
------------------------------------------------------------------------------
Omnibus: 0.000 Durbin-Watson: 2.000
Normal (N): 1.127 Prob(N): 0.569
==============================================================================
Note that JB, P(JB), skew and kurtosis have different values.
Note that Prob(Q) tests no correlation of residuals.
8 # Import.
9 import sys
10 import warnings
11 import pandas as pd
12 import matplotlib as mpl
13 import matplotlib.pyplot as plt
14
15 # Import sarimax
16 from statsmodels.tsa.statespace.sarimax import SARIMAX
17
18
19 # import weights.
20 from pyamr.datasets.load import make_timeseries
21 from pyamr.core.regression.sarimax import SARIMAXWrapper
22
23 # Filter warnings
24 warnings.simplefilter(action='ignore', category=FutureWarning)
25
26 # ----------------------------
27 # set basic configuration
28 # ----------------------------
29 # Matplotlib options
30 mpl.rc('legend', fontsize=6)
31 mpl.rc('xtick', labelsize=6)
32 mpl.rc('ytick', labelsize=6)
33
34 # Set pandas configuration.
35 pd.set_option('display.max_colwidth', 14)
36 pd.set_option('display.width', 150)
37 pd.set_option('display.precision', 4)
38
39 # ----------------------------
40 # create data
41 # ----------------------------
42 # Create timeseries data
43 x, y, f = make_timeseries()
44
45 # Create exogenous variable
46 exog = x
47
48 # ----------------------------
49 # fit the model
50 # ----------------------------
51 # Create specific sarimax model.
52 sarimax = SARIMAXWrapper(estimator=SARIMAX)\
53 .fit(endog=y[:80], exog=None, trend='ct',
54 seasonal_order=(1,0,1,12), order=(0,1,1),
55 disp=0)
56
57
58 # Print series
59 print("\nSeries:")
60 print(sarimax.as_series())
61
62 # Print summary.
63 print("\nSummary:")
64 print(sarimax.as_summary())
65
66 # -----------------
67 # Save & Load
68 # -----------------
69 # File location
70 #fname = '../../examples/saved/arima-sample.pickle'
71
72 # Save
73 #arima.save(fname=fname)
74
75 # Load
76 #arima = ARIMAWrapper().load(fname=fname)
77
78
79 # -----------------
80 # Predict and plot
81 # -----------------
82 # This example shows how to make predictions using the wrapper which has
83 # been previously fitted. It also demonstrateds how to plot the resulting
84 # data for visualization purposes. It shows two different types of
85 # predictions:
86 # - dynamic predictions in which the prediction is done based on the
87 # previously predicted values. Note that for the case of ARIMA(0,1,1)
88 # it returns a line.
89 # - not dynamic in which the prediction is done based on the real
90 # values of the time series, no matter what the prediction was for
91 # those values.
92
93 # Variables.
94 s, e = 50, 120
95
96 # Compute predictions
97 preds_1 = sarimax.get_prediction(start=s, end=e, dynamic=False)
98 preds_2 = sarimax.get_prediction(start=s, end=e, dynamic=True)
99
100 # Create figure
101 fig, axes = plt.subplots(1, 2, figsize=(8,3))
102
103 # ----------------
104 # Plot non-dynamic
105 # ----------------
106 # Plot truth values.
107 axes[0].plot(y, color='#A6CEE3', alpha=0.5, marker='o',
108 markeredgecolor='k', markeredgewidth=0.5,
109 markersize=5, linewidth=0.75, label='Observed')
110
111 # Plot forecasted values.
112 axes[0].plot(preds_1[0,:], preds_1[1,:], color='#FF0000', alpha=1.00,
113 linewidth=2.0, label=sarimax._identifier())
114
115 # Plot the confidence intervals.
116 axes[0].fill_between(preds_1[0,:], preds_1[2,:],
117 preds_1[3,:],
118 color='#FF0000',
119 alpha=0.25)
120
121 # ------------
122 # Plot dynamic
123 # ------------
124 # Plot truth values.
125 axes[1].plot(y, color='#A6CEE3', alpha=0.5, marker='o',
126 markeredgecolor='k', markeredgewidth=0.5,
127 markersize=5, linewidth=0.75, label='Observed')
128
129 # Plot forecasted values.
130 axes[1].plot(preds_2[0,:], preds_2[1,:], color='#FF0000', alpha=1.00,
131 linewidth=2.0, label=sarimax._identifier())
132
133 # Plot the confidence intervals.
134 axes[1].fill_between(preds_2[0,:], preds_2[2,:],
135 preds_2[3,:],
136 color='#FF0000',
137 alpha=0.25)
138
139 # Configure axes
140 axes[0].set_title("ARIMA non-dynamic")
141 axes[1].set_title("ARIMA dynamic")
142
143 # Format axes
144 axes[0].grid(True, linestyle='--', linewidth=0.25)
145 axes[1].grid(True, linestyle='--', linewidth=0.25)
146
147 # Legend
148 axes[0].legend()
149 axes[1].legend()
150
151 # Show
152 plt.show()
Total running time of the script: ( 0 minutes 0.501 seconds)