Note
Go to the end to download the full example code
Metrics - weights
Example to show how to use the SigmoidA
class to compute weights.
Todo
The SigmoidA
class name stands for Sigmoid Approximation.
Probably this example should include external references, a
better explanation of the sigmoid function and how the SigmoidA
(approximation) is computed and how to use it.
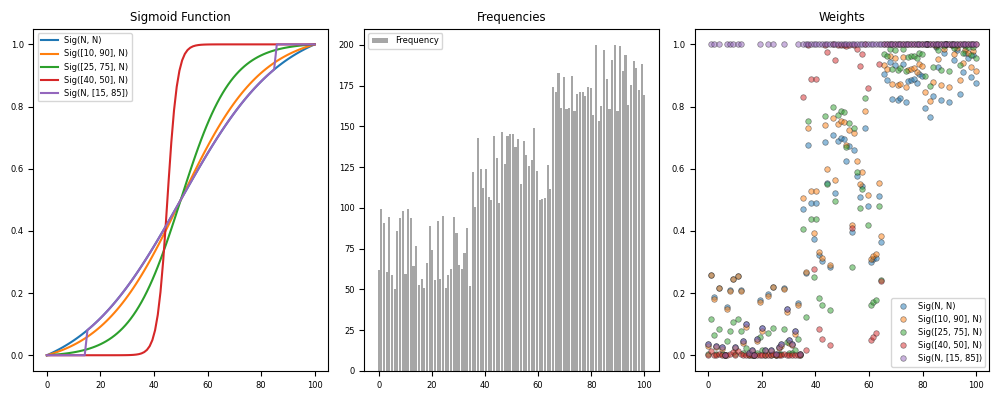
12 # Libraries
13 import numpy as np
14 import pandas as pd
15 import matplotlib as mpl
16 import matplotlib.pyplot as plt
17 import statsmodels.robust.norms as norms
18
19 # pyAMR
20 from pyamr.metrics.weights import SigmoidA
21
22 # Matplotlib options
23 mpl.rc('legend', fontsize=6)
24 mpl.rc('xtick', labelsize=6)
25 mpl.rc('ytick', labelsize=6)
26 mpl.rc('font', size=7)
27
28 # Set pandas configuration.
29 pd.set_option('display.max_colwidth', 14)
30 pd.set_option('display.width', 150)
31 pd.set_option('display.precision', 4)
32
33 # Constants
34 length = 100
35
36 # Create time-series.
37 x = np.linspace(0,100,100)
38 f = np.concatenate((np.random.rand(35)*50+50,
39 np.random.rand(30)*50+100,
40 np.random.rand(35)*50+150))
41
42 # Weight Functions
43 w_functions = [
44 SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0),
45 SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0, percentiles=[10,90]),
46 SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0, percentiles=[25,75]),
47 SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0, percentiles=[40,50]),
48 SigmoidA(r=200, g=0.5, offset=0.0, scale=1.0, thresholds=[15,85])
49 ]
50
51 # Create figure
52 fig, axes = plt.subplots(1,3, figsize=(10,4))
53
54 # Plot frequencies
55 axes[1].bar(x, f, color='gray', alpha=0.7, label='Frequency')
56
57 # Plot weights
58 for i,W in enumerate(w_functions):
59 axes[0].plot(x, W.weights(x), label=W._identifier(short=True))
60 axes[2].plot(x, W.weights(f), marker='o', alpha=0.5,
61 markeredgecolor='k', markeredgewidth=0.5, markersize=4,
62 linewidth=0.00, label=W._identifier(short=True))
63
64 # Titles
65 axes[0].set_title('Sigmoid Function')
66 axes[1].set_title('Frequencies')
67 axes[2].set_title('Weights')
68
69 # Legends
70 axes[0].legend()
71 axes[1].legend()
72 axes[2].legend()
73
74 # Tight layout
75 plt.tight_layout()
76
77 # Show
78 plt.show()
Total running time of the script: ( 0 minutes 0.354 seconds)