Note
Click here to download the full example code
Sample 01ΒΆ
This examples computes the three most basic standard SQIs (kurtosis, skewness and entropy). In addition, it shows the code in a one big section. It is just an example.
Warning
The dtw
module seems to be loaded even though
we are not really using it. Instead, load it
only when required.
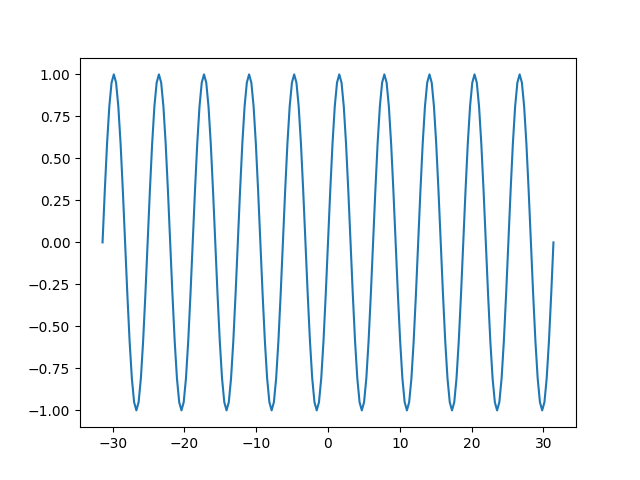
Out:
Result:
kurtosis -1.492500e+00
skewness -1.605493e-16
entropy 4.997679e+00
dtype: float64
15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | # Libraries generic
import numpy as np
import pandas as pd
# Libraries specific
from vital_sqi.sqi.standard_sqi import perfusion_sqi
from vital_sqi.sqi.standard_sqi import kurtosis_sqi
from vital_sqi.sqi.standard_sqi import skewness_sqi
from vital_sqi.sqi.standard_sqi import entropy_sqi
from vital_sqi.sqi.standard_sqi import signal_to_noise_sqi
# ---------------------------
# Main
# ---------------------------
# Create samples
x = np.linspace(-10*np.pi, 10*np.pi, 201)
# Create signal
signal = np.sin(x)
# Loop computing sqis
k = kurtosis_sqi(signal)
s = skewness_sqi(signal)
e = entropy_sqi(signal)
# Create Series
result = pd.Series(
data=[k, s, e],
index=['kurtosis', 'skewness', 'entropy']
)
# Show
print("\nResult:")
print(result)
# ---------------
# Create plot
# ---------------
# Library
import matplotlib.pyplot as plt
# Plot
plt.plot(x, signal)
# Show
plt.show()
|
Total running time of the script: ( 0 minutes 0.074 seconds)